In this Blog we are going to talk about Mastering Node.js Modules: The Key to Efficient, Scalable Cod eIn today’s fast-paced world, learning to write scalable, maintainable, and efficient code is critical, especially in Node.js, which has become one of the most popular JavaScript runtime environments. If you’ve already read our Getting Started with Node.js post, you can set up your Node.js environment and run basic scripts.
It’s time to take your skills to the next level by mastering Node.js modules.
if you want to master node.js you can download the Bootself app and start learning from beginner to advanced level.
Why should you care? Modules are at the heart of every Node.js project. They enable you to organize your code, keep it clean, and make it reusable. Whether you’re building a small app or working on a larger-scale project, understanding modules is essential.
If you want a more hands-on learning experience, try our AI-enabled BootSelf app, which helps you learn coding through real-time feedback. Our AI mentor provides explanations, suggestions, and code reviews—available 24/7 to fit your pace and goals.
What are Node.js Modules?
In Mastering Node.js: The Node.js module is essentially a block of reusable code that encapsulates functionality, making your app more maintainable. Imagine modules like small, well-defined building blocks you can combine to create larger, more complex applications.
Node.js allows you to use built-in modules, custom modules, and npm packages, offering flexibility and scalability in your app development process.
- What are the built-in modules in Node.js?
Node.js comes with a variety of built-in modules such as:fs
: Used for interacting with the file system.http
: Helps create web servers.path
: Simplifies path manipulation.
You can easily access these modules using the require()
function in your project.
- How do I create a custom module in Node.js?
To create a custom module, simply define your code in one file and export it usingmodule.exports
. For example, create a modulemath.js
:javascript
module.exports = { add };function add(a, b) {
return a + b;
}
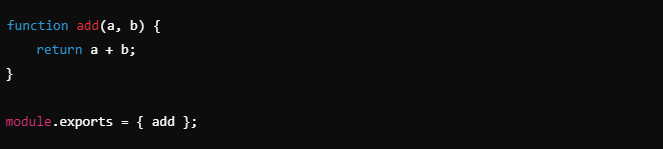
Then, import it into another file using require()
:
const math = require('./math');
console.log(math.add(5, 3)); // Outputs 8
- What are npm packages, and how do I use them in Node.js?
npm packages are external modules you can install using npm (Node Package Manager). They help add functionalities like HTTP requests, data validation, and more. To use a package likeaxios
:- Run
npm install axios
in your terminal - Use it in your code:
- Run
- How can I organize large Node.js applications with modules?
In large projects, dividing functionality into different modules is essential for maintainability. By breaking down code into reusable parts, you ensure that each module handles a single responsibility. You can also group related modules into directories to maintain structure. For example:/models
for data models/controllers
for logic/routes
for defining endpoints
You can take your learning further with BootSelf, where you can build projects and receive real-time feedback from an AI mentor.
Built-in and External Modules in Node.js
Node.js comes with a variety of built-in modules that allow you to perform different functions without the need for third-party libraries. Here are a few examples:
fs
: For file system operationshttp
: To create web serverspath
: For file path manipulation
For external modules, npm is your go-to tool. You can find thousands of pre-built libraries in the npm registry. Here’s how to install and use lodash
, a popular utility library:
- Install the package:
bash
npm install lodash
- Use the package in your application:
javascript
const _ = require('lodash');
let numbers = [10, 5, 2, 8];
let sortedNumbers = _.sortBy(numbers);
console.log(sortedNumbers); // [2, 5, 8, 10]
To get a better feel for these concepts, use BootSelf, where our AI mentor provides personalized guidance as you work through real-world coding problems.
How to Create Custom Modules in Node.js
To demonstrate how to create and use custom modules, let’s go through an example:
- Create a file named
math.js
:javascript
function divide(a, b) {function multiply(a, b) {
return a * b;
}
if (b === 0) throw new Error(‘Division by zero is not allowed’);
return a / b;
}Custom Modules in Node.js module.exports = { multiply, divide };
- Use the
math.js
module inapp.js
:
Breaking your code into reusable modules allows you to maintain clean code, even as your project grows.
Use BootSelf for Interactive Node.js Learning
If you’re looking to take your Node.js skills further, the BootSelf AI-powered coding app is here to help. Whether you’re struggling with a tricky concept or need real-time code reviews, BootSelf offers:
- 24/7 AI Mentor: Available to answer any coding questions.
- Real-time Code Reviews: Get immediate feedback on your coding practices.
- Code Generation: Use AI to generate code snippets aligned with best practices.
- Custom Learning Pathways: Practice at your own pace, aligning with your goals and level.
BootSelf helps you bridge the gap between learning and real-world coding challenges. Download today to start coding smarter, not harder.
Now that you have a solid grasp of how to work with modules in Node.js, you’re ready to start building more complex and scalable applications. Mastering Node Modules requires a practical and guided way to achieve it you can download the Bootself app to master 50+ tranding languages Whether it’s organizing your codebase using custom modules or leveraging npm packages, mastering this core concept is a crucial step in becoming a Node.js pro.
If you have an interview coming up and are looking for quick support on MERN you can go to this post
Stay tuned for our next blog post, where we’ll explore asynchronous programming in Node.js and how to handle multiple tasks efficiently. Meanwhile, don’t forget to check out BootSelf, your personal AI coding mentor, for 24/7 support and guidance!
Master Your Coding Skills with BootSelf AI
If you're looking to enhance your coding abilities and upskill in artificial intelligence, look no further than the BootSelf AI app. This innovative platform provides AI-based coding lessons that are tailored to your individual learning pace.
Available on both iOS and Android, you can download the BootSelf AI app and start mastering coding skills today: